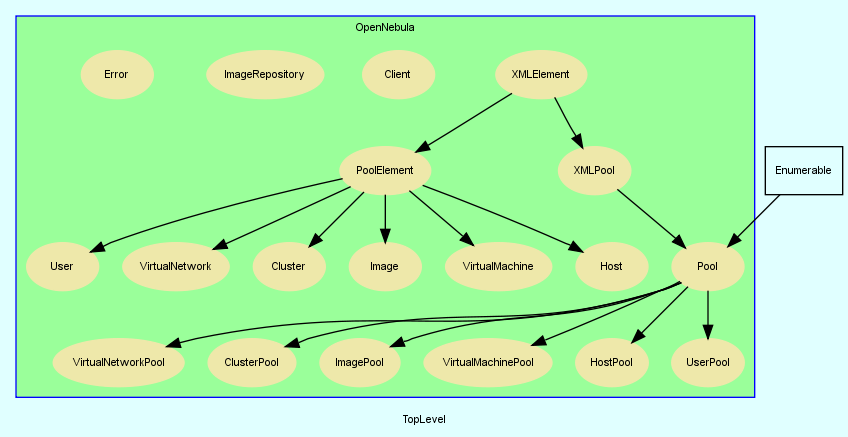
Methods
public class
public instance
Constants
IMAGE_METHODS | = | { :info => "image.info", :allocate => "image.allocate", :update => "image.update", :rmattr => "image.rmattr", :enable => "image.enable", :publish => "image.publish", :persistent => "image.persistent", :delete => "image.delete" } |
Constants and Class Methods |
|
IMAGE_STATES | = | %w{INIT READY USED DISABLED} | ||
SHORT_IMAGE_STATES | = | { "INIT" => "init", "READY" => "rdy", "USED" => "used", "DISABLED" => "disa" } | ||
IMAGE_TYPES | = | %w{OS CDROM DATABLOCK} | ||
SHORT_IMAGE_TYPES | = | { "OS" => "OS", "CDROM" => "CD", "DATABLOCK" => "DB" } |
Public class methods
Creates an Image description with just its identifier this method should be used to create plain Image objects. id the id of the image
Example:
image = Image.new(Image.build_xml(3),rpc_client)
# File OpenNebula/Image.rb, line 60 60: def Image.build_xml(pe_id=nil) 61: if pe_id 62: image_xml = "<IMAGE><ID>#{pe_id}</ID></IMAGE>" 63: else 64: image_xml = "<IMAGE></IMAGE>" 65: end 66: 67: XMLElement.build_xml(image_xml,'IMAGE') 68: end
Class constructor
# File OpenNebula/Image.rb, line 71 71: def initialize(xml, client) 72: super(xml,client) 73: 74: @client = client 75: end
Public instance methods
Allocates a new Image in OpenNebula
description A string containing the template of the Image.
# File OpenNebula/Image.rb, line 89 89: def allocate(description) 90: super(IMAGE_METHODS[:allocate],description) 91: end
Deletes the Image
# File OpenNebula/Image.rb, line 140 140: def delete() 141: super(IMAGE_METHODS[:delete]) 142: end
Disables an Image
# File OpenNebula/Image.rb, line 115 115: def disable 116: set_enabled(false) 117: end
Enables an Image
# File OpenNebula/Image.rb, line 110 110: def enable 111: set_enabled(true) 112: end
Retrieves the information of the given Image.
# File OpenNebula/Image.rb, line 82 82: def info() 83: super(IMAGE_METHODS[:info], 'IMAGE') 84: end
Makes the Image non persistent
# File OpenNebula/Image.rb, line 135 135: def nonpersistent 136: set_persistent(false) 137: end
Makes the Image persistent
# File OpenNebula/Image.rb, line 130 130: def persistent 131: set_persistent(true) 132: end
Publishes the Image, to be used by other users
# File OpenNebula/Image.rb, line 120 120: def publish 121: set_publish(true) 122: end
Deletes an Image attribute
name Name of the attribute to be deleted
# File OpenNebula/Image.rb, line 105 105: def remove_attr(name) 106: do_rm_attr(name) 107: end
Returns the state of the Image (string value)
# File OpenNebula/Image.rb, line 160 160: def short_state_str 161: SHORT_IMAGE_STATES[state_str] 162: end
Returns the state of the Image (string value)
# File OpenNebula/Image.rb, line 175 175: def short_type_str 176: SHORT_IMAGE_TYPES[type_str] 177: end
Returns the state of the Image (numeric value)
# File OpenNebula/Image.rb, line 150 150: def state 151: self['STATE'].to_i 152: end
Returns the state of the Image (string value)
# File OpenNebula/Image.rb, line 155 155: def state_str 156: IMAGE_STATES[state] 157: end
Returns the type of the Image (numeric value)
# File OpenNebula/Image.rb, line 165 165: def type 166: self['TYPE'].to_i 167: end
Returns the type of the Image (string value)
# File OpenNebula/Image.rb, line 170 170: def type_str 171: IMAGE_TYPES[type] 172: end
Unplubishes the Image
# File OpenNebula/Image.rb, line 125 125: def unpublish 126: set_publish(false) 127: end
Modifies an image attribute
name Name of the attribute to be changed
value New value for the attribute
# File OpenNebula/Image.rb, line 98 98: def update(name, value) 99: super(IMAGE_METHODS[:update], name, value) 100: end